알고리즘
프로그래머스 전화번호 목록 (Python)
풀이 참조: https://youtu.be/4-iyppqNCyg https://programmers.co.kr/learn/courses/30/lessons/42577 코딩테스트 연습 - 전화번호 목록 전화번호부에 적힌 전화번호 중, 한 번호가 다른 번호의 접두어인 경우가 있는지 확인하려 합니다. 전화번호가 다음과 같을 경우, 구조대 전화번호는 영석이의 전화번호의 접두사입니다. 구조 programmers.co.kr 위 유튜브 영상을 보고 정리하였습니다. 개발자로 취직하기 님께 감사드립니다. Solution 1 이중포문을 쓰고 if 문 2개로 서로 접두어인지 확인한다. 테스트케이스 2개가 시간초과가 뜬다. # Loop를 활용한 솔루션 : 시간초과 뜸. def solution_Loop(phone_book): ..
LeetCode 88. Merge Sorted Array (Python)
https://leetcode.com/problems/merge-sorted-array/ Merge Sorted Array - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com Solution 1 시간복잡도 : O(NlongN) 공간복잡도 : O(N) 아이디어 (정렬) 아주 단순하다. nums1 뒤에 넣고 정렬을 한다. 그리고 일반 할당이 아닌 슬라이스 할당을 하였다. 이렇게 하면 참조(주소)에 의한 호출로 동작하므로 값이 바뀐다. # 1. 정렬 def merge(..
LeetCode 35.Search Insert Position (Python)
https://leetcode.com/problems/search-insert-position/ Search Insert Position - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com Solution 1 시간복잡도 : O(N) 공간복잡도 : O(1) 아이디어 (Brute- Force) 배열을 0에서부터 순회하고, target의 값과 같거나 크다면 순회 중단하고 중단한 시점의 인덱스를 반환한다. # 1. Brute force def searchInsert(nu..
LeetCode 26. Remove Duplicates From Sorted Array (Python)
https://leetcode.com/problems/remove-duplicates-from-sorted-array/ Remove Duplicates from Sorted Array - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com Solution 시간복잡도: O(n) 공간복잡도: O(1) 아이디어(Brute-Force) 중복이 없는 상태의 길이를 반환하라는 문제인데, 단순히 중복되지 않는 수의 갯수를 세서 이를 반환하는 식으로 구함. .. 이라고 생각했는데,..
LeetCode 1. Two Sum (Python)
https://leetcode.com/problems/two-sum/ Two Sum - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com Solution 1 Brute Force 시간복잡도 : O(n^2) 공간복잡도 : O(1) def twoSum(nums: List[int], target: int) -> List[int]: for i in range(len(nums)): for j in range(i+1, len(nums)): if (nums[i] + nums[j..
LeetCode 66 Plus One
https://leetcode.com/problems/plus-one/ Plus One - LeetCode Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview. leetcode.com 풀이 참조: https://the-dev.tistory.com/55 public int[] plusOne(int[] digits) { int carry =1 ; int index = digits.length -1; while (index >= 0 && carry > 0) { digits[index] = (digits[index..
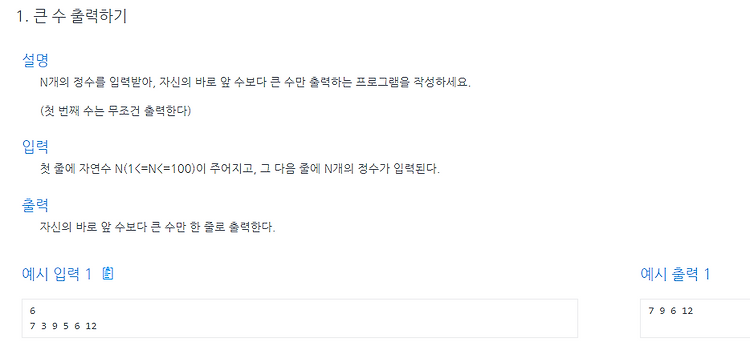
인프런 13. 큰 수 출력하기 (Java)
내 풀이 import java.util.Scanner; public class Main { public void solution(int[] arr) { StringBuilder sb = new StringBuilder(); sb.append(arr[0]).append(" "); for (int i = 0; i < arr.length- 1; i++) { if (arr[i] < arr[i+1]) { sb.append(arr[i+1]).append(" "); } } System.out.println(sb); } public static void main(String[] args) { Main T = new Main(); Scanner sc = new Scanner(System.in); int n = sc.ne..
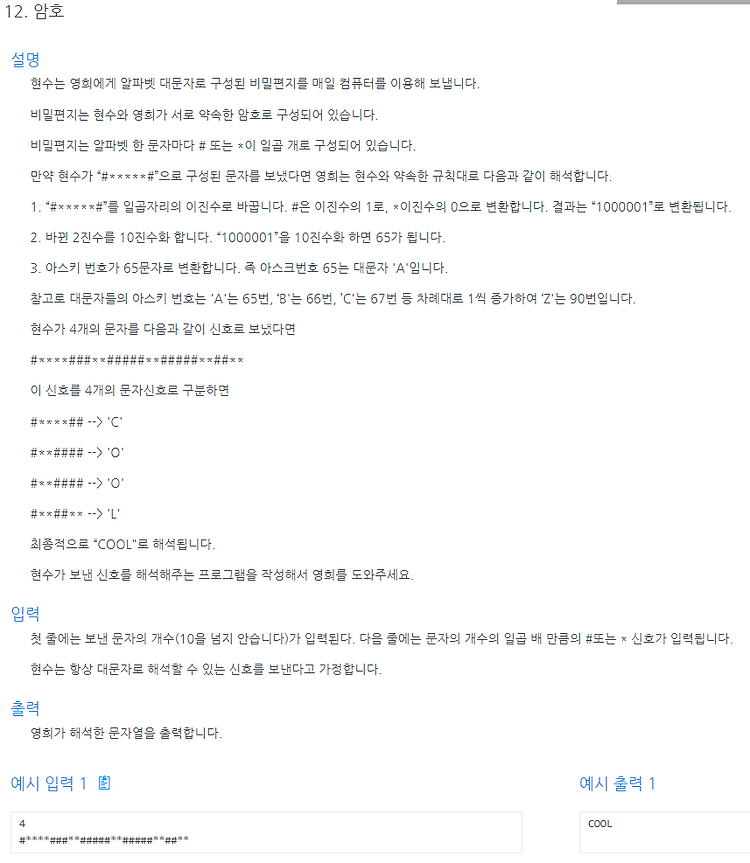
인프런 12. 암호(JAVA)
풀이 어려워 보이고 하다가 잘 안되어서 풀이 봤는데 너무 쉽게 풀어서 황당함. substring으로 7개 끊고, replace로만 # -> 1로 * -> 0으로 바꾸고 Integer.parseInt로 2진수 10진수로 변환하고 그 아스키 문자를 char로 형변환하여 answer에 붙여준다. 마지막으로 substring으로 끊어서 7개 이후의 문자로 다시 for문을 돈다. import java.util.Scanner; public class Main { private String solution(int n, String s) { String answer = ""; for (int i = 0; i < n; i++) { String tmp = s.substring(0, 7).replace('#', '1').r..
코드업 1916 : (재귀함수) 피보나치 수열 (Large)
피보나치 수열이란 앞의 두 수를 더하여 나오는 수열이다. 첫 번째 수와 두 번째 수는 모두 1이고, 세 번째 수부터는 이전의 두 수를 더하여 나타낸다. 피보나치 수열을 나열해 보면 다음과 같다. 1, 1, 2, 3, 5, 8, 13 … 자연수 N을 입력받아 N번째 피보나치 수를 출력하는 프로그램을 작성하시오. 단, N이 커질 수 있으므로 출력값에 10,009를 나눈 나머지를 출력한다. ※ 이 문제는 반드시 재귀함수를 이용하여 작성 해야한다. 금지 키워드 : for while goto 입력 자연수 N이 입력된다. (N은 200보다 같거나 작다.) 출력 N번째 피보나치 수를 출력하되, 10,009를 나눈 나머지 값을 출력한다. 입력 예시 7 출력 예시 13 풀이 DP를 처음 배울 때 많이 나오는 풀이 방법으..
코드업 1403 배열 두번 출력하기
https://codeup.kr/problem.php?id=1403 배열 두번 출력하기 k개의 숫자를 입력받은 순서대로 한 줄에 하나씩 출력한다. 그리고 한번 출력이 다 되면 다시 한번더 출력한다.(총 2번) codeup.kr 내 풀이 배열 두 번 출력하도록 2번 도는 외부 포문안에 배열 전체를 도는 for문을 배치하고. \n으로 한 줄 씩 출력하게 하였다. public class Codeup1403 { public static void main(String[] args) throws IOException { BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); BufferedWriter bw = new BufferedWrit..